Adding "EnhancedInput" module to Build.cs
https://docs.unrealengine.com/5.0/en-US/API/Plugins/EnhancedInput/UEnhancedInputEngineSubsystem/

Create a new character class ,
add "InputActionValue.h" to header of this new character class to recognize FInputActionValue return type, https://docs.unrealengine.com/5.2/en-US/API/Plugins/EnhancedInput/FInputActionValue/
#include "InputActionValue.h"

forward declare UInputMappingContext and UInputAction in the header
class UInputMappingContext;
class UInputAction;

create two pointers in protected section for MappingContext and InputAction so we can add them in the editor to blueprint version of the character
and create a callback function for specific action
protected:
virtual void BeginPlay() override;
UPROPERTY(EditAnywhere, BlueprintReadOnly , Category = Input)
UInputMappingContext* CharacterMappingContext;
UPROPERTY(EditAnywhere, BlueprintReadOnly , Category = Input)
UInputAction* MoveInputAction;
void MoveCallBack(const FInputActionValue& Value);
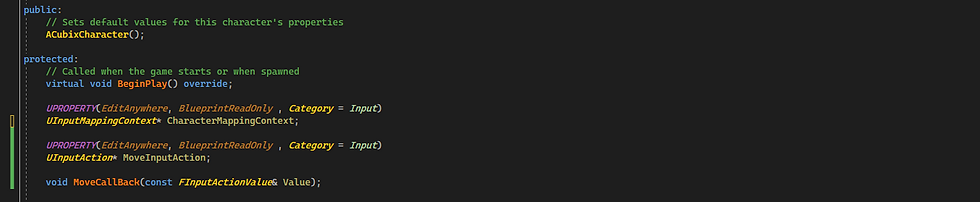
Done with header,
In c++ part add these two header

in the BeginPlay() we need to fill the mappingContext using this method:
find player controller, and find enhanced subsystem from localplayer static function "GetSubsystem()"
then using AddMappingContext functon to define the mappingContext
void ACubixCharacter::BeginPlay()
{
Super::BeginPlay();
if (APlayerController* PlayerController = Cast<APlayerController>(GetController()))
{
UEnhancedInputLocalPlayerSubsystem* EnhancedSubsystem = ULocalPlayer::GetSubsystem<UEnhancedInputLocalPlayerSubsystem>(PlayerController->GetLocalPlayer());
if (EnhancedSubsystem)
{
EnhancedSubsystem->AddMappingContext(CharacterMappingContext, 0);
}
}
}

for binding action in SetupPlayerInputComponent(),
make a pointer to UEnhancedInputComponent and use it to bind actions,
// Called to bind functionality to input
void ACubixCharacter::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent)
{
Super::SetupPlayerInputComponent(PlayerInputComponent);
if (UEnhancedInputComponent* EnhancedInputComponent = CastChecked<UEnhancedInputComponent>(PlayerInputComponent))
{
EnhancedInputComponent->BindAction(MoveInputAction, ETriggerEvent::Triggered, this, &ACubixCharacter::MoveCallBack);
}
}
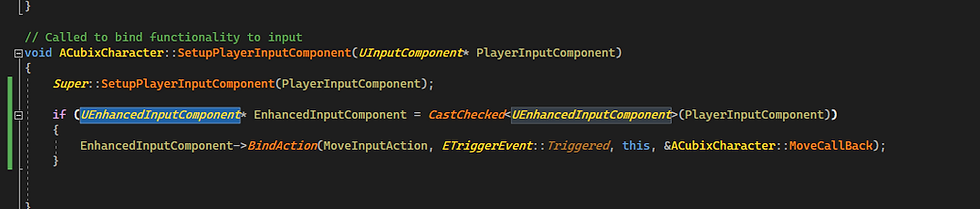
the rest is expanding it by adding input actions , creating callback function and linking them together in cpp ,
void ACubixCharacter::MoveCallBack(const FInputActionValue& Value)
{
const FVector MoveVector = Value.Get<FVector>();
UE_LOG(LogTemp, Warning , TEXT("value is %f , %f , %f"), MoveVector.X , MoveVector.Y , MoveVector.Z)
}

in editor create a blueprint of the created class and add mappingContext and Inputactions and assign it.
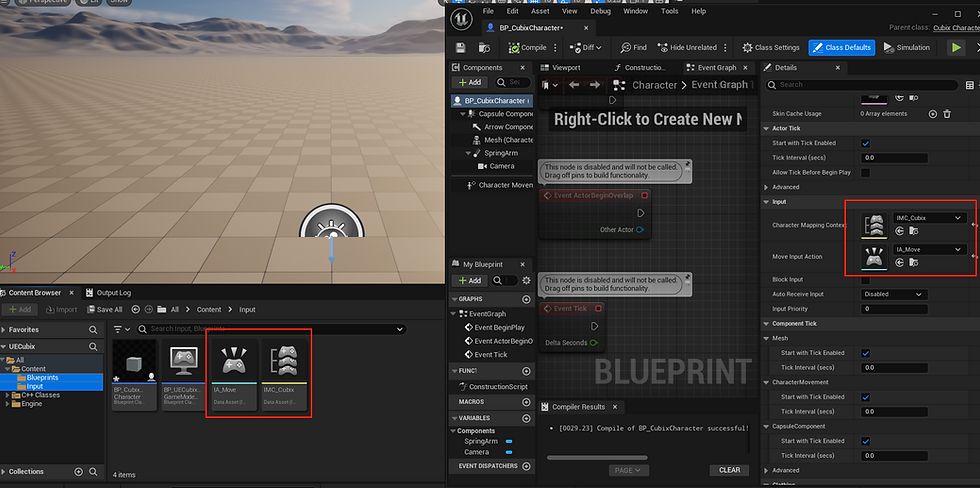
Comments